The Model Context Protocol (MCP) is a powerful standard for connecting Large Language Models (LLMs) to external data and tools, acting like a universal bridge for AI interactions. If you’re a Python developer looking to enhance your AI workflows, FastMCP is the go-to library to simplify building MCP servers. In this blog, we’ll walk through setting up a basic MCP server using FastMCP, complete with a practical example to get you started.
Whether you’re new to MCP or a seasoned developer, this guide will help you create a server that enables LLMs to interact with custom tools and data. Let’s dive in!
Want to get started quickly?
If you know python well, and you can “Click” and scroll to “Copy-and-Paste Example” section.
What is FastMCP?
FastMCP is a Pythonic, high-level framework that abstracts the complexities of the MCP protocol, such as server setup, protocol handlers, and error management. It allows you to focus on building tools and resources for LLMs using simple Python functions and decorators. Think of it as a way to make your Python code AI-ready with minimal effort.
What is MCP? The Model Context Protocol (MCP) is a set of rules that lets AI models, like LLMs, connect to external data and tools. Use MCP when you need your AI to:
- Fetch External Data: Query databases or APIs (e.g., weather updates or sales figures).
- Run Custom Tools: Execute tasks you’ve programmed, like calculations or file editing.
- Enhance AI Capabilities: Combine AI with your systems for practical, real-world results.
For example, MCP can let an AI add two numbers using a tool you’ve built or pull a personalised greeting from a custom resource; similarly, you can create your own tools or integrations to empower AI with unique capabilities tailored to your needs. Even a documentation search MCP?
Prerequisites
Before we start, ensure you have:
- Python 3.7+ installed on your system.
- A terminal (macOS/Linux) or PowerShell/CMD (Windows).
- Basic familiarity with Python and virtual environments.
- (Optional) An MCP-compatible client like Claude Desktop or Cursor for testing.
Step 1: Install FastMCP
The recommended way to install FastMCP is using uv, a fast Python package manager that simplifies dependency management. If you’re new to Python, uv is a tool that makes it easy to install libraries (pre-written code) and manage project environments. It’s faster and simpler than traditional tools like pip
and virtualenv
, combining their features into one command. With uv, you can quickly set up projects and ensure consistent results with minimal hassle.
If you don’t have uv installed, you can install it via:
# On macOS/Linux
curl -LsSf https://astral.sh/uv/install.sh | sh
# On Windows (PowerShell)
powershell -c "irm https://astral.sh/uv/install.ps1 | iex"
After installing uv, create a project directory and set up a virtual environment:
mkdir my-mcp-server
cd my-mcp-server
uv init
uv venv
source .venv/bin/activate # On Windows: .venv\Scripts\activate
Now, install FastMCP with the optional CLI tools for debugging:
uv pip install "mcp[cli]"
Verify the installation by checking the FastMCP version:
fastmcp version
You should see output like:
FastMCP version: 0.4.2.dev41+ga077727.d20250410
MCP version: 1.6.0
Python version: 3.12.2
Step 2: Create a Simple MCP Server
Let’s build a basic MCP server with a tool that adds two numbers. Create a file named server.py
in your project directory and add the following code:
from mcp.server.fastmcp import FastMCP
# Initialize the MCP server with a name
mcp = FastMCP("CalculatorServer")
# Define a tool to add two numbers
@mcp.tool()
def add(a: int, b: int) -> int:
"""Add two numbers and return the result."""
return a + b
# Run the server
if __name__ == "__main__":
mcp.run(transport="stdio")
This code:
- Imports
FastMCP
from the MCP library. - Creates a server instance named “CalculatorServer”.
- Defines a tool called
add
using the@mcp.tool()
decorator, which allows an LLM to call this function. - Runs the server using the
stdio
transport, ideal for local testing.
Step 3: Test the Server with MCP Inspector
FastMCP includes a handy debugging tool called MCP Inspector, which provides a UI to test your server without connecting to an LLM client. Start the server in development mode:
uv run mcp dev server.py
This command launches the server and opens the MCP Inspector in your browser (typically at http://127.0.0.1:6274
).

If it doesn’t open automatically, navigate to that URL. Once loaded:
- Click Connect to link to your server.
- In the Tools tab, you’ll see the
add
tool listed. - Click on it, enter values (e.g.,
a=
4,b=3
), and select Run Tool to test. You should see the result: 7.

Step 4: Integrate with an LLM Client (Optional)
To see your server in action with an LLM, integrate it with an MCP-compatible client like Claude Desktop or Cursor. Here’s how to set it up with Cursor:
- Open your project directory in Cursor.
- Go to File → Preferences → Cursor Settings → MCP → Add New Server.
- Configure the server:
- Name: “Calculator”
- Type: Command
- Command:
uv run mcp run /path/to/your/server.py
- Save the configuration. A green 🟢 indicator should appear, confirming the server is running.
- In Cursor’s chat interface, type: “Add 5 and 3.” The LLM should call your
add
tool and return8
.
If you encounter a 🟠 indicator, double-check the file path and ensure the virtual environment is activated.
Step 5: Expand Your Server
Now that you have a working server, you can add more tools or resources. For example, let’s add a resource to greet users:
# Add to server.py
@mcp.resource("greeting://{name}")
def get_greeting(name: str) -> str:
"""Return a personalized greeting."""
return f"Hello, {name}!"
Restart the server with uv run mcp dev server.py
, and test the resource in MCP Inspector by accessing greeting://John
to see “Hello, John!” You can also call this resource from Cursor by asking, “Get a greeting for John.”
Copy-and-Paste Example
Want to get started quickly? Below is a complete, ready-to-run FastMCP server script that includes both the add
tool and the greeting
resource. Simply click the “Copy” button (or select and copy the code) and paste it into a file named server.py
.
from mcp.server.fastmcp import FastMCP
# Initialize the MCP server
mcp = FastMCP("MyFirstMCPServer")
# Define a tool to add two numbers
@mcp.tool()
def add(a: int, b: int) -> int:
"""Add two numbers and return the result."""
return a + b
# Define a resource for personalized greetings
@mcp.resource("greeting://{name}")
def get_greeting(name: str) -> str:
"""Return a personalized greeting."""
return f"Hello, {name}!"
# Run the server
if __name__ == "__main__":
mcp.run(transport="stdio")
How to Run:
- Click the “Copy” button above (or select and copy the code) and save it as
server.py
in your project directory. - Ensure FastMCP is installed (
uv pip install "mcp[cli]"
). - Activate your virtual environment (
source .venv/bin/activate
or.venv\Scripts\activate
on Windows). - Run the server:
uv run mcp dev server.py
. - Open
http://127.0.0.1:6274
in your browser to test with MCP Inspector. Try theadd
tool (e.g.,a=10
,b=20
) or thegreeting
resource (e.g.,greeting://Alice
).
This script is a fully functional starting point you can build upon!
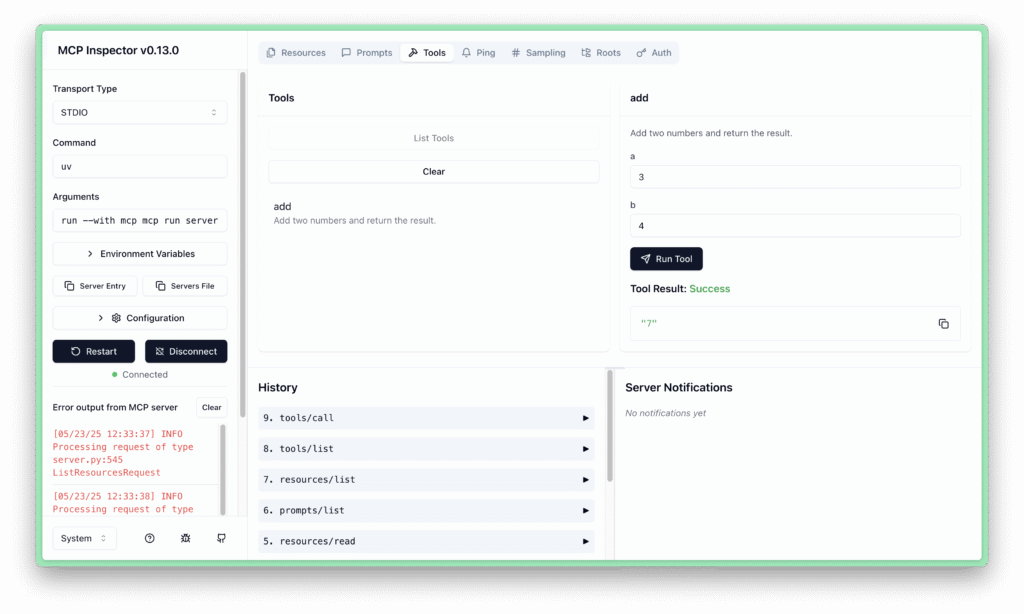

Why Use FastMCP?
FastMCP simplifies MCP server development with:
- Minimal Boilerplate: Create tools and resources with just a few lines of code.
- Pythonic Design: Uses decorators and type hints for a familiar experience.
- Powerful Features: Supports async functions, OpenAPI integration, and client libraries for advanced use cases.
- Debugging Tools: MCP Inspector makes testing straightforward.
Next Steps
You’ve now set up a basic MCP server with FastMCP! From here, you can:
- Add more complex tools, like querying APIs or databases (e.g., SQLite or yfinance).
- Deploy your server to a cloud platform like AWS or Cloudflare.
- Explore the FastMCP documentation for advanced features like server proxying or LLM sampling.
To dive deeper, check out the FastMCP GitHub repository or the official MCP Python SDK. Start building smarter AI workflows today!
Happy coding, and enjoy powering your LLMs with FastMCP!